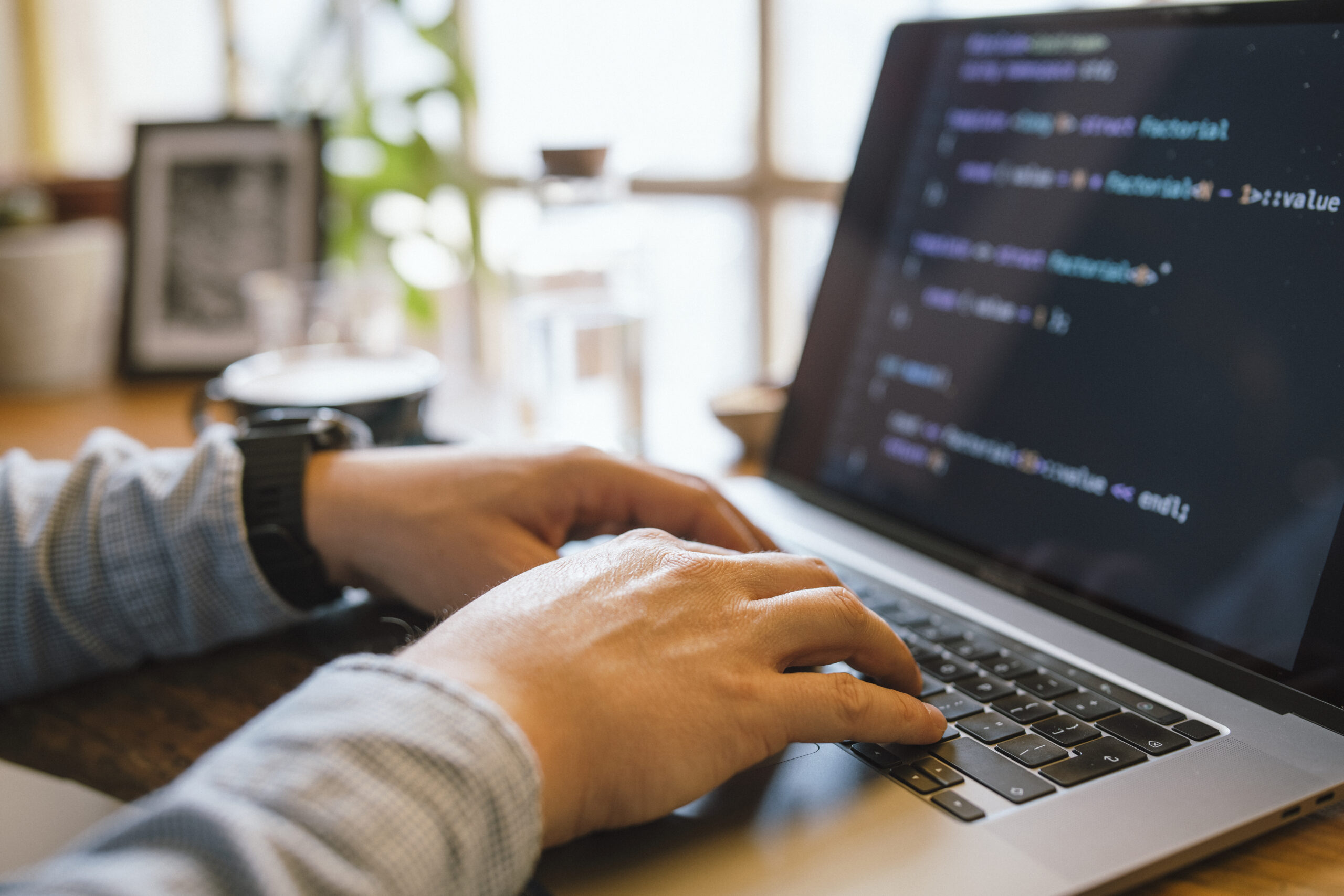
Debugging is Among the most critical — however typically overlooked — expertise in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Studying to Feel methodically to resolve difficulties proficiently. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productiveness. Listed below are numerous approaches to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest methods builders can elevate their debugging competencies is by mastering the instruments they use every single day. Although writing code is one Component of growth, realizing how you can connect with it proficiently in the course of execution is equally significant. Present day improvement environments occur Geared up with effective debugging capabilities — but quite a few developers only scratch the area of what these equipment can perform.
Just take, for instance, an Built-in Advancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code about the fly. When used effectively, they let you notice exactly how your code behaves through execution, that is a must have for tracking down elusive bugs.
Browser developer instruments, such as Chrome DevTools, are indispensable for front-close developers. They help you inspect the DOM, keep track of community requests, view actual-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can flip annoying UI issues into manageable jobs.
For backend or procedure-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle about running processes and memory management. Discovering these tools might have a steeper Mastering curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Further than your IDE or debugger, grow to be relaxed with Variation Manage techniques like Git to be aware of code record, discover the exact second bugs ended up launched, and isolate problematic improvements.
Finally, mastering your tools indicates going past default settings and shortcuts — it’s about building an intimate familiarity with your progress atmosphere in order that when concerns come up, you’re not misplaced at midnight. The higher you recognize your equipment, the more time you'll be able to devote solving the actual problem rather than fumbling through the procedure.
Reproduce the condition
One of the more significant — and infrequently missed — ways in productive debugging is reproducing the challenge. Ahead of jumping into the code or making guesses, builders need to have to make a constant atmosphere or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug will become a match of opportunity, often bringing about wasted time and fragile code modifications.
The initial step in reproducing a challenge is gathering as much context as is possible. Request concerns like: What actions brought about The difficulty? Which ecosystem was it in — growth, staging, or creation? Are there any logs, screenshots, or error messages? The greater depth you've, the easier it will become to isolate the exact disorders beneath which the bug occurs.
As soon as you’ve collected plenty of info, seek to recreate the challenge in your local setting. This might necessarily mean inputting precisely the same data, simulating related person interactions, or mimicking technique states. If The difficulty appears intermittently, take into consideration creating automatic tests that replicate the edge scenarios or state transitions concerned. These checks not only enable expose the problem but in addition reduce regressions Later on.
At times, The difficulty may be setting-unique — it might take place only on selected operating methods, browsers, or underneath individual configurations. Utilizing equipment like Digital machines, containerization (e.g., Docker), or cross-browser screening platforms can be instrumental in replicating this sort of bugs.
Reproducing the situation isn’t simply a stage — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But after you can continuously recreate the bug, you're currently halfway to repairing it. That has a reproducible scenario, You should use your debugging resources far more proficiently, exam potential fixes safely, and talk far more Plainly with the staff or buyers. It turns an summary criticism right into a concrete obstacle — Which’s where by builders prosper.
Read and Comprehend the Error Messages
Error messages are sometimes the most useful clues a developer has when a thing goes Completely wrong. Rather than viewing them as aggravating interruptions, developers ought to learn to treat mistake messages as immediate communications from your program. They frequently tell you exactly what transpired, wherever it transpired, and in some cases even why it transpired — if you understand how to interpret them.
Commence by studying the information thoroughly and in full. Lots of developers, especially when underneath time strain, glance at the first line and promptly start out generating assumptions. But deeper inside the error stack or logs may perhaps lie the real root result in. Don’t just duplicate and paste error messages into engines like google — study and have an understanding of them initially.
Break the mistake down into elements. Is it a syntax error, a runtime exception, or perhaps a logic mistake? Does it position to a specific file and line variety? What module or function activated it? These questions can information your investigation and point you towards the liable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Discovering to recognize these can greatly accelerate your debugging process.
Some problems are imprecise or generic, As well as in Individuals conditions, it’s vital to look at the context during which the error transpired. Look at connected log entries, enter values, and up to date changes within the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede bigger troubles and supply hints about probable bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, encouraging you pinpoint concerns more rapidly, lower debugging time, and become a much more effective and assured developer.
Use Logging Properly
Logging is The most highly effective applications in a developer’s debugging toolkit. When utilized properly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood without having to pause execution or move in the code line by line.
A fantastic logging tactic commences with figuring out what to log and at what stage. Widespread logging stages incorporate DEBUG, Details, Alert, ERROR, and Deadly. Use DEBUG for thorough diagnostic data all through enhancement, Facts for normal gatherings (like thriving start-ups), WARN for potential issues that don’t crack the appliance, ERROR for real problems, and Lethal if the technique can’t carry on.
Steer clear of flooding your logs with too much or irrelevant facts. Excessive logging can obscure crucial messages and slow down your procedure. Center on critical activities, state improvements, input/output values, and critical final decision points in the code.
Structure your log messages clearly and continuously. Involve context, which include timestamps, ask for IDs, and performance names, so it’s easier to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Permit you to observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all devoid of halting the program. They’re Primarily useful in production environments wherever stepping by means of code isn’t attainable.
In addition, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, smart logging is about equilibrium and clarity. Using a well-imagined-out logging approach, it is possible to lessen the time it will take to identify challenges, acquire deeper visibility into your apps, and improve the In general maintainability and reliability of the code.
Imagine Like a Detective
Debugging is not simply a technical undertaking—it's a type of investigation. To properly detect and repair bugs, developers have to tactic the procedure similar to a detective solving a mystery. This attitude will help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the indications of the condition: mistake messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, gather as much pertinent details as you'll be able to with no jumping to conclusions. Use logs, check circumstances, and user reviews to piece together a transparent photograph of what’s going on.
Upcoming, kind hypotheses. Question oneself: What could possibly be leading to this behavior? Have any changes not too long ago been created towards the codebase? Has this issue happened in advance of beneath equivalent circumstances? The intention will be to slim down prospects and identify opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the issue in a managed surroundings. If you suspect a selected operate or element, isolate it and validate if The problem persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Fork out close notice to modest particulars. Bugs generally conceal during the minimum envisioned spots—like a missing semicolon, an off-by-one error, or a race issue. Be thorough and client, resisting the urge to patch the issue with no fully comprehension it. Temporary fixes may possibly disguise the true challenge, only for it to resurface later on.
Last of all, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future troubles and assistance Other individuals fully grasp your reasoning.
By thinking like a detective, builders can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering hidden concerns in advanced units.
Create Exams
Composing assessments is among the simplest methods to boost your debugging capabilities and In general development efficiency. Tests not just support capture bugs early and also function a security Web that offers you assurance when making changes for your codebase. A effectively-tested application is easier to debug because it permits you to pinpoint specifically where by and when a dilemma takes place.
Get started with device assessments, which center on particular person features or modules. These modest, isolated assessments can speedily reveal regardless of whether a particular piece of logic is working as expected. Any time a exam fails, you promptly know wherever to seem, drastically lowering time spent debugging. Device assessments are Specifically helpful for catching regression bugs—problems that reappear after Beforehand currently being set.
Subsequent, combine integration assessments and stop-to-finish checks into your workflow. These support make certain that numerous aspects of your software function alongside one another efficiently. They’re specifically useful for catching bugs that come about in sophisticated techniques with multiple parts or solutions interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Feel critically about your code. To test a feature appropriately, you'll need to be aware of its inputs, expected outputs, and edge scenarios. This degree of knowledge Normally sales opportunities to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. When the exam fails constantly, you may concentrate on repairing the bug and check out your check move when The difficulty is fixed. This method makes sure that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing video game right into a structured and predictable procedure—supporting you capture extra bugs, faster and even more reliably.
Just take Breaks
When debugging a tough difficulty, it’s easy to become immersed in the trouble—watching your display screen for several hours, seeking Alternative following Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your head, lower irritation, and infrequently see The difficulty from a new standpoint.
If you're too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you choose to wrote just several hours previously. In this particular condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a unique process for 10–15 minutes can refresh your focus. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially all through more time debugging periods. Sitting down before a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed Power in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just check here before.
If you’re caught, a good guideline would be to established a timer—debug actively for 45–sixty minutes, then have a 5–ten minute split. Use that point to move all-around, stretch, or do a thing unrelated to code. It might sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually brings about faster and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is part of fixing it.
Study From Every Bug
Every single bug you come upon is more than just a temporary setback—It truly is a possibility to grow as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each can educate you anything important if you take some time to mirror and examine what went Erroneous.
Get started by inquiring you a few important queries after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code testimonials, or logging? The solutions usually reveal blind spots inside your workflow or comprehending and enable you to Construct more powerful coding routines moving forward.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log where you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or widespread blunders—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug with your friends might be Specifically powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other individuals avoid the similar concern boosts team effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers will not be the ones who publish perfect code, but individuals who continuously understand from their mistakes.
Ultimately, Each individual bug you resolve provides a brand new layer to the talent set. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills will take time, exercise, and patience — nevertheless the payoff is big. It will make you a more productive, self-confident, and capable developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.